Scripting
Bonkstrap has added some JavaScript APIs for adding functionality to your page that can't (or shouldn't) be done in just CSS. Functionality is divided into separate files, so each page can just include scripts that it needs. All modules (they aren't real modules though :( ) are bundled into a single object in the global namespace, Bonkstrap
.
Modals
A modal is a sub-window within an interface. Specifically, a modal requires that the user interact with it before they can continue to use the parent interface. The modal puts the parent interface into a disabled mode.
Modal dialogs are not supported in Firefox; all dialogs there are modeless.
Modal with header and small content
Bonkstrap.createModal('Foo', '<p>hello world</p>');
Modal with header and large content
Bonkstrap.createModal('Foo', big_text);
Modal with expandable details
Bonkstrap.createModalDetails('Expandable information', 'The quick brown fox jumped over the candlestick, knocking it over and ultimately causing the Great Fire of London.');
Modal with just small content
Bonkstrap.createModalRaw('<p>hello world</p>');
Modal with just large content
Bonkstrap.createModalRaw(big_text);
Modal with arbitrary HTML content
Note that this example doesn't work well on smaller screens. We can make the framework responsive, but your content is your responsibility.
Bonkstrap.createModalRaw(map_embed);
Slideshow
An image slideshow is a collection of images that scroll to display one at a time. To create a slideshow, wrap a series of img
tags in a container, and give that container the data-bonk-slideshow
attribute. It is recommended to assign the container a fixed height as well, but this is a matter of preference. So long as you specify a height, Bonkstrap does a stand-up job of handling very differently-sized images in the same slideshow. The frog below is much larger than the watermelon lady, and buff Barney is in a portrait aspect ratio.
<div data-bonk-slideshow class="height-20rem">
<img src="images/scripting/frog.jpg"/>
<img src="images/scripting/bernie.jpg"/>
<img src="images/scripting/despacito.jpg"/>
<img src="images/scripting/watermelone.jpeg"/>
<img src="images/scripting/orang-man-attak.jpg"/>
</div>
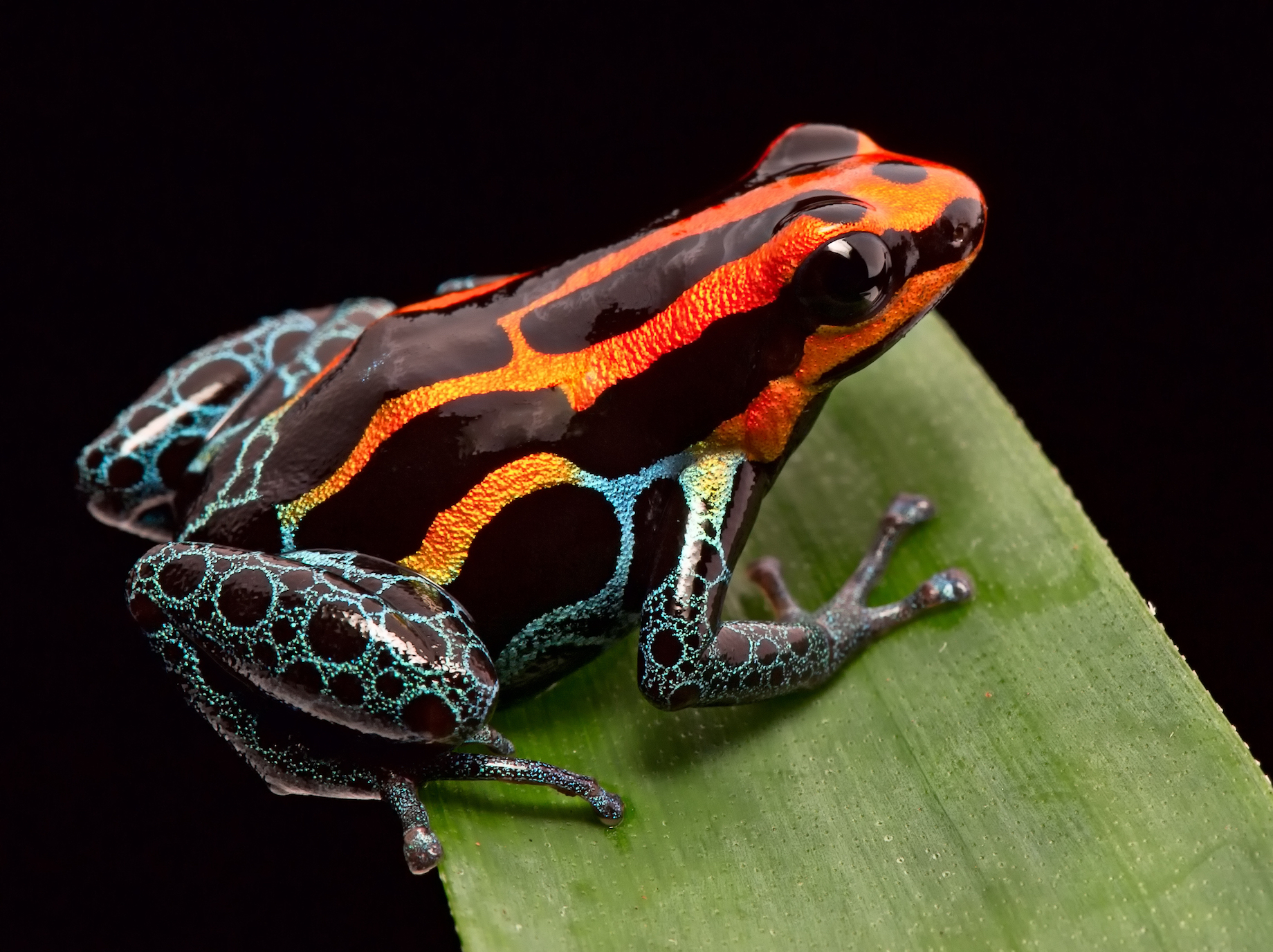
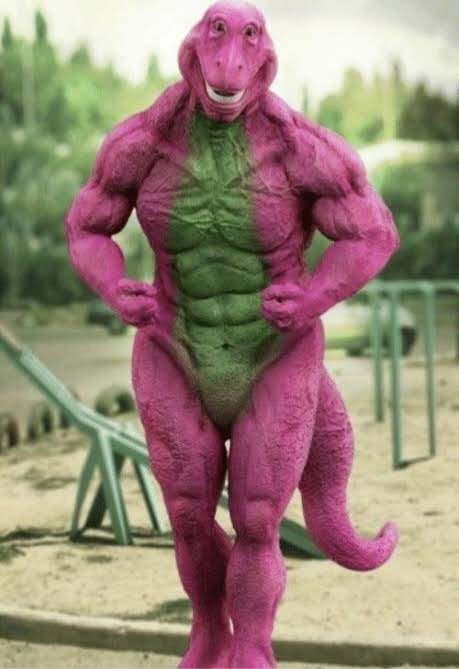
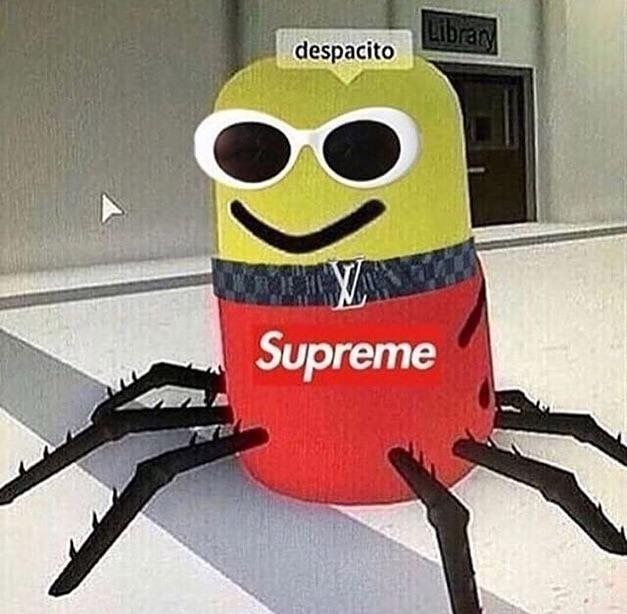
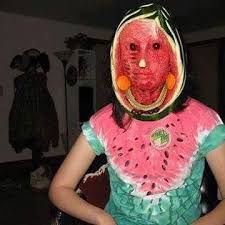
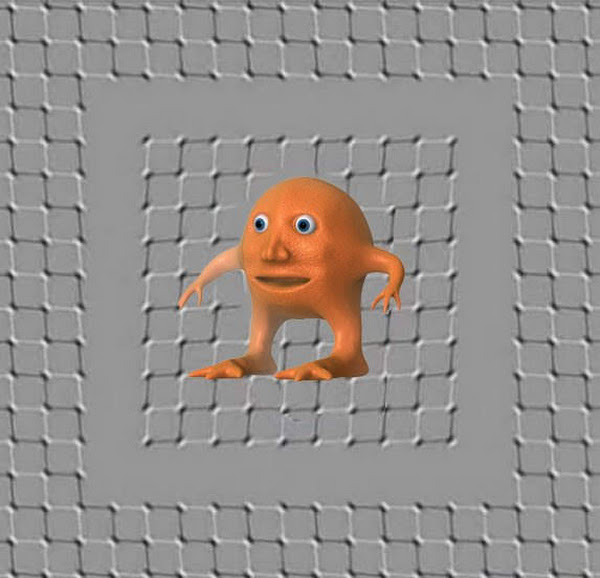
Message of the Day
The "message of the day" is a concept dating back at least as far as 1984 (see Multics documentation). When users logged into a system, they would be presented with a sitewide but administrator-configurable message, which was more efficient than sending mass emails.
Bonkstrap supports the automatic filling of identified elements with arbitrary content returned from a (possibly asynchronous) function call or expression—think MOTD but in the general case. To mark an element for this treatment, give it the data-bonk-motd
attribute, and assign to it a string to evaluate to obtain the desired content. Any functions or variables referenced should be defined by the time DOMContentLoaded
is fired. Anything that was in the element will be removed and replaced with the new content when it arrives. If the evaluation throws an error, the content will not be updated. MOTD elements may be refreshed at any time by calling Bonkstrap.updateMOTDs()
, or individually via something like Bonkstrap.motdRecpts[n].update()
, where n
is the index of the desired MOTD element. Bonkstrap.motdRecpts
is a regular array of Bonkstrap.classes.MOTDRecipient
s. If you wish to dive further, you are welcome to do so by exploring the source. It's not that bad, I promise.
<div data-bonk-motd="simAPICall()">Content visible immediately at <code>DOMContentLoaded</code></div>
DOMContentLoaded